Code Examples
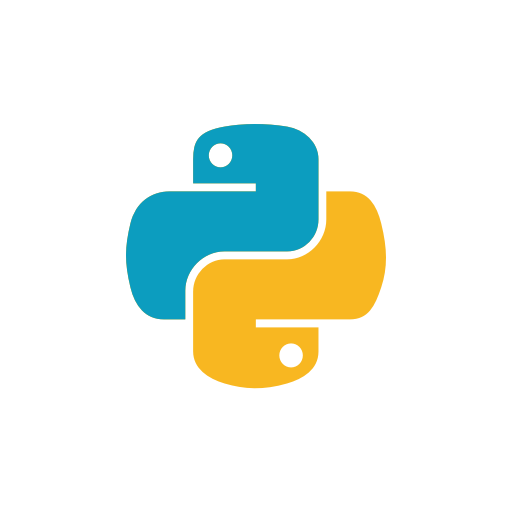
Python examples
.
Simple motion towards a target position
import socket import time # Configure Socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to motor s.connect(('192.168.1.103', 1000)) # Send control command s.sendall(b'"<control pos=\"1000\" speed=\"500\" torque=\"200\" mode=\"129\" acc=\"1000\" decc=\"1000\" />"') time.sleep(5)
Oscillation: y = A * cos( f * t + B) + C
import socket import time # Configure Oscillation # y = Amplitude * cos( frequency * t + phase) + offset gearRatio = 50; # ratio if no speed reducer then set to 1 amplitude = 30; # amplitude in degrees frequency = 20; # frequency in 1/100 * 1/s phase = 0; # phase offset in 1/10 radiant offset = 0; # horizontal offset in degrees # Configure Socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect to motor s.connect(('192.168.1.102', 1000)) # Compose String to send to the drive st = '"<control pos=\"' + str(gearRatio*amplitude*10) + '\" frequency=\"' + str(frequency) + '\" torque=\"200\" mode=\"135\" offset=\"' + str(gearRatio*offset*10) + '\" phase=\"' + str(phase) + '\" />"'; s.sendall(st.encode('ascii')) # send XML command # wait 15 seconds before demo ends time.sleep( 15 )
Send and receive commands
import socket import struct import threading import time import signal def tcp_send_command_thread(stop_event): # Configure Socket with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s: # Connect to motor s.connect(('192.168.1.102', 1000)) # Compose String to send to the drive st = '"<control pos=\"15000\" frequency=\"20\" torque=\"200\" mode=\"135\" offset=\"0\" phase=\"0\" />"'
s.sendall(st.encode('ascii')) # send XML command # The main thread can continue executing other tasks while not stop_event.is_set(): time.sleep(1) def receive_udp_data(udp_port, stop_event): # Configure Socket with socket.socket(socket.AF_INET, socket.SOCK_DGRAM) as s: s.bind(('', udp_port)) while not stop_event.is_set(): data, addr = s.recvfrom(132) # buffer size is 132 bytes int_list = list(struct.unpack("<33i", data)) # interprete 33 int32 numbers # Print the list print('time:' + str(int_list[0]) + ' position:' + str(int_list[1]) + ' speed:' + str(int_list[2])) def keyboard_interrupt_handler(signal, frame): print("Keyboard interrupt received. Stopping the program.") # Set the stop event to signal the thread to exit stop_event.set() # Exit the program exit(0) if __name__ == '__main__': stop_event = threading.Event() # create an event object # create a thread that receives on UDP port 1001 udp_receive_thread = threading.Thread(target=receive_udp_data, args=(1001, stop_event)) udp_receive_thread.start() # create a thread that can send commands to the motor tcp_send_command_thread = threading.Thread(target=tcp_send_command_thread, args=(stop_event,)) tcp_send_command_thread.start() # Install the keyboard interrupt handler signal.signal(signal.SIGINT, keyboard_interrupt_handler) # The main thread can continue executing other tasks while True: # Do other stuff here time.sleep(1)
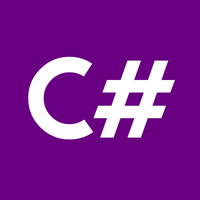
C# example
A finished C# driver is available at GitHub
using System; using System.Net.Sockets; using System.Text; namespace ConsoleApplication { class Program { static void Main(string[] args) { //create instance from TCPClient Object var client = new TcpClient("192.168.1.102", 1000); //if a client has found, the program continues Console.WriteLine("client found!"); //create an instance of the C# Stream class and get the Stream to the client var networkStream = client.GetStream(); byte[] encodedBytes = System.Text.Encoding.ASCII.GetBytes("<control pos=\"1000\" speed=\"500\" torque=\"200\" mode=\"129\" acc=\"1000\" decc=\"1000\" />"); //send the drive order to the HDrive networkStream.Write(encodedBytes, 0, encodedBytes.Length); //continuously read data comming from the HDrive byte[] buffer = new byte[85]; // read in chunks of 85 Byte int bytesRead; while ((bytesRead = networkStream.Read(buffer, 0, buffer.Length)) > 0) { Console.WriteLine(Encoding.Default.GetString(buffer)); } } } }
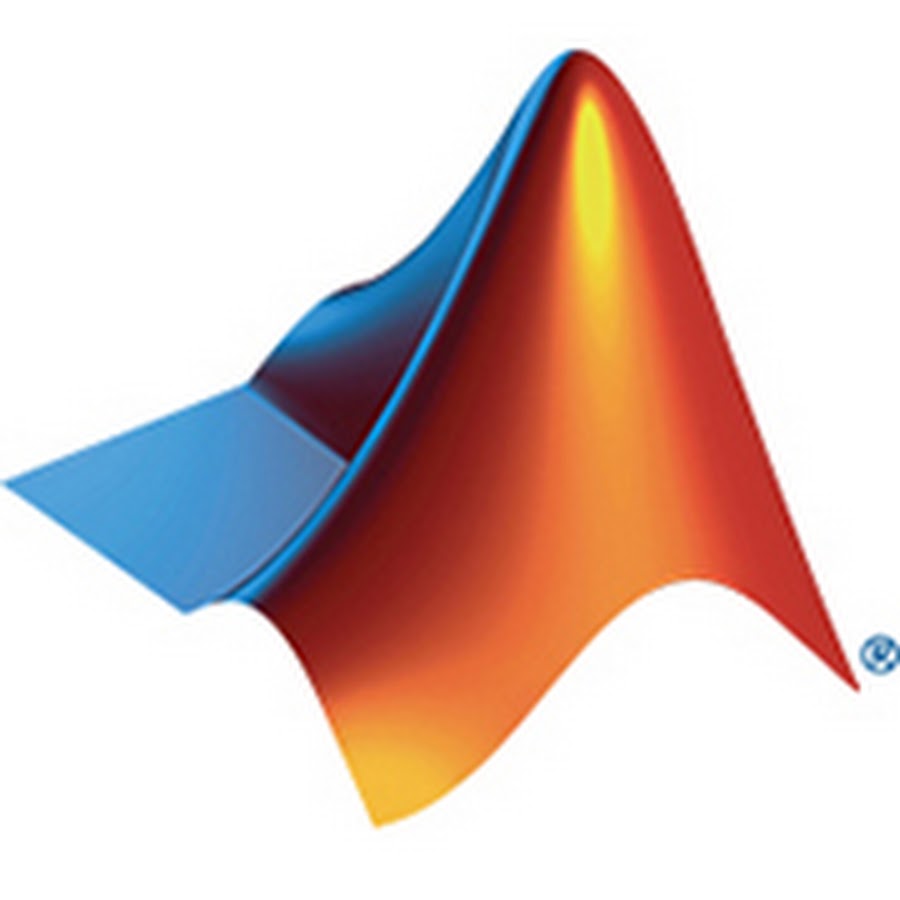
Matlab example
Send HDrive ticket
t=tcpip('192.168.1.102', 1000, 'NetworkRole', 'client'); %configure TCP Client and Port fopen(t); % open TCP Connection fwrite(t, '<control pos=\"1000\" speed=\"500\" torque=\"200\" mode=\"129\" acc=\"1000\" decc=\"1000\" />') % send XML command pause( 5 ) % wait 5 seconds (the motor would stop if you close the connection before) fclose(t);
Receive HDrive ticket (TCP mode)
Please notice: The HDrive is sending the Tickets with up to 2 kHz. This means you have to read very fast to always get the newest ticket. Therefore it is recommended to read the ticket in a loop or to reduce the send frequency by setting up a pre-scaler with the web interface.
clear all; t=tcpip('192.168.1.102', 1000, 'NetworkRole', 'client'); %configure TCP Client and Port fopen(t);%open TCP Connection A = fread(t, 150); %receive one HDrive ticket % convert received bytes into string recieved_string = sprintf(' % interpret string time_ms = str2num(recieved_string(137:146)); position = str2num(recieved_string(23:32)); calibration = str2num(recieved_string(59:62)); speed_RPM = str2num(recieved_string(42:49)); % close connection fclose(t);
Receive HDrive ticket (UDP mode)
In this scenario, the HDrive has to be configured to send UDP tickets to the host. This configuration can be done in the Web interface
hold on; clear all; %configure and open UDP Connection u=udp('192.168.1.102','RemotePort',2000,'Localport',1001); set(u,'DatagramTerminateMode','off'); u.ByteOrder = 'littleEndian'; fopen(u); % read UDP data and convert to 32bit integers data = fread(u, 3, 'int32'); %store data to local variables time = data(1); position = data(2); speed = data(3); % close connection fclose(u);
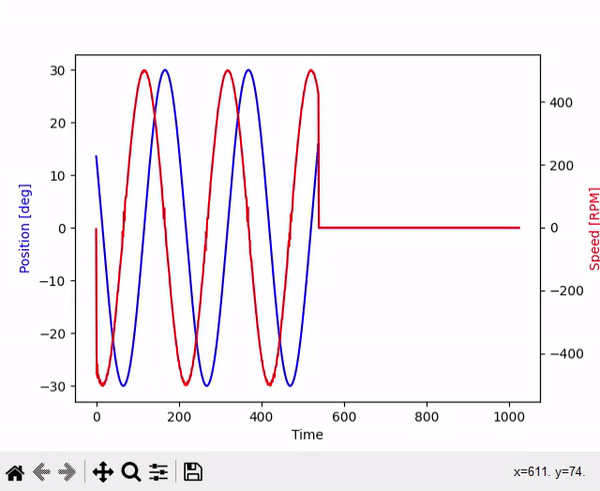
Demo application - Python and live graph
This is a short Demo written in Python to show how to drive the motor with Python and also on how to receive data from the drive like position, time or speed
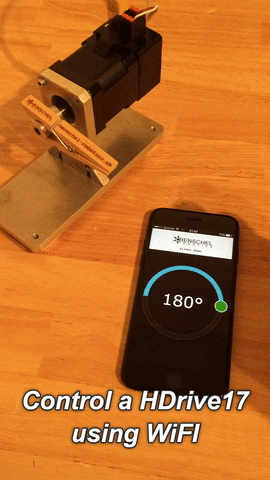
Demo application - Drive and mobile phone
This is a short Demo written in JavaScript on how to control a HDrive. The code is executed inside a web browser running on a local web server or on the HDrive entirely.
The web page is only containing a circular slider and some lines of JavaScript code to control the HDrive. This page can then be hosted almost anywhere.
It then is possible to access the page with the circular slider from a wireless device if everything is setup properly.